moveForward
moveForward() is the easiest way to move the turtle in a straight line in the direction it is facing. When used with turnLeft() and turnRight() you can navigate the turtle anywhere or draw any straight line pictures.
Examples
Basic Example
// Move forward 25 pixels (default)
moveForward();
Example: Letter L
Draw the letter L with moveForward() and turnLeft() only.
// Draw the letter L with moveForward() and turnLeft() only.
turnLeft();
turnLeft();
moveForward();
moveForward();
turnLeft();
moveForward();
Example: Long Line
Move forward 200 pixels.
// Move forward 200 pixels
moveForward(200);
Example: Lemon Popsicle
Draw a lemon popsicle by changing the pen color and width, and by specifing how many pixels the turtle should move in the direction forwards and backwards.
// Draw a lemon popsicle by changing the pen color and width
// and by specifing how many pixels the turtle should move in the direction forwards and backwards.
penColor("yellow");
penWidth(40);
moveForward(100);
penWidth(5);
penColor("brown");
moveForward(-200);
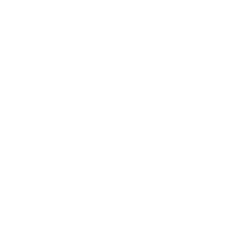
Syntax
moveForward(pixels);
Parameters
Name | Type | Required? | Description |
---|---|---|---|
pixels | number | The number of pixels to move the turtle forward in its current direction. If not provided, the turtle will move forward 25 pixels |
Returns
Tips
- Use penUp() before calling moveForward() to stop the turtle from drawing a line behind it as it moves.
- The screen default size is 320 pixels wide and 450 pixels high, but you can move the turtle off the screen by exceeding those dimensions.
- There are three ways to move the turtle in a straight line:
- Specify the number of pixels to move the turtle in the direction it is facing using moveForward(pixels) or moveBackward(pixels).
- Specify a number of pixels in the x and y direction to move the turtle using move(x,y), regardless of direction that the turtle is facing.
- Specify an x and y pixel location on the screen to move the turtle to using moveTo(x,y), regardless of direction that the turtle is facing.
Found a bug in the documentation? Let us know at support@code.org.