Painter
Fields
Type | Name | Description |
---|---|---|
int | xLocation | the x coordinate of the |
int | yLocation | the y coordinate of the |
String | direction | the direction the |
int | remainingPaint | the number of units of paint the |
Method Details
Painter
public Painter()
Creates a Painter
object at (0, 0)
facing "East"
with 0
units of paint
Examples
Painter myPainter = new Painter();
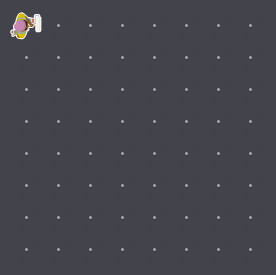
Painter
public Painter(int x, int y, String direction, int paint)
Creates a Painter
at specific x and y coordinates facing a specified direction with a given number of units of paint.
Parameters
Name | Type | Description |
---|---|---|
x | int | the x coordinate to place the |
y | int | the y coordinate to place the |
direction | String | the direction for the |
paint | int | the number of units of paint the |
Examples
Painter myPainter = new Painter(2, 4, "South", 10);
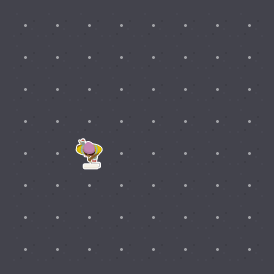
move
public void move()
Moves the Painter
object one space forward in the direction it is facing.
Examples
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter.move();
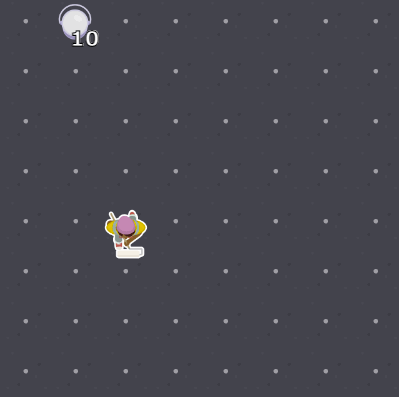
turnLeft
public void turnLeft()
Turns a Painter
object to the left.
Examples
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter .move();
myPainter .turnLeft();
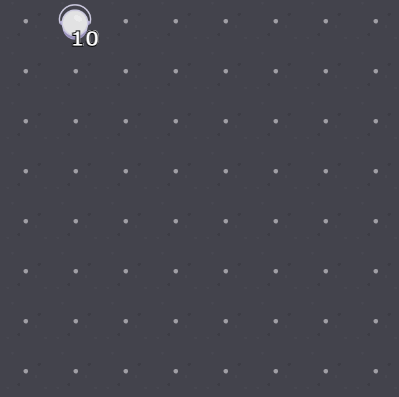
paint
public void paint(String color)
Paints the space the Painter
object is standing on.
Parameters
Name | Type | Description |
---|---|---|
color | String | the color of the paint - can be a color name or a hex value |
Examples
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter.paint("white");
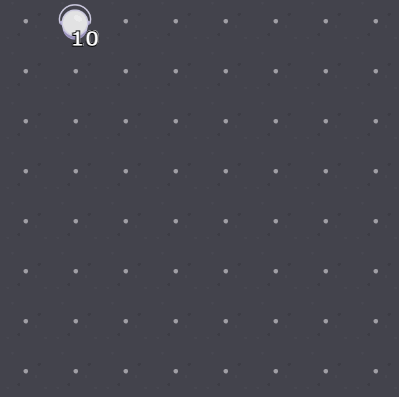
Color Options
takePaint
public void takePaint()
Takes paint from the paint bucket the Painter
object is currently standing on and adds a single unit of paint to their paint bucket. The number of units of paint in the paint bucket decreases by 1
. If the Painter
object is not on a paint bucket, nothing happens.
Examples
Painter myPainter = new Painter();
myPainter.move();
myPainter.takePaint();
myPainter.move();
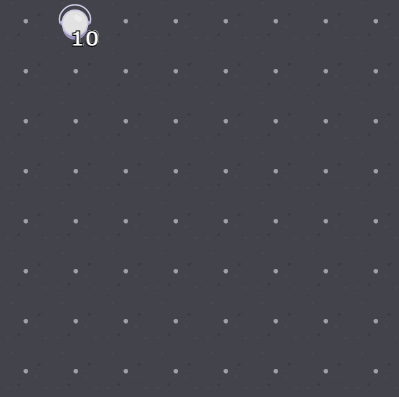
scrapePaint
public void scrapePaint()
Removes the paint from the space the Painter
object is standing on.
Examples
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter.paint("white");
myPainter.move();
myPainter.paint("white");
myPainter.turnLeft();
myPainter.turnLeft();
myPainter.move();
b.scrapePaint();
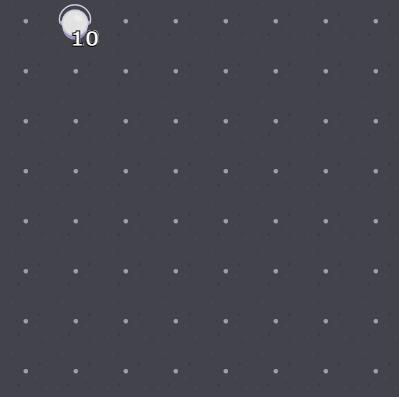
canMove
public boolean canMove()
Returns true
if there is no barrier one space ahead in the direction the Painter
object is currently facing.
Examples
canMove() Returns false
Painter myPainter = new Painter(2, 3, "east", 0);
boolean moveStatus = myPainter.canMove();
System.out.println("Painter can move forward: " + moveStatus);
Output
Painter can move forward: false
canMove() Returns true
Painter myPainter = new Painter(2, 3, "east", 0);
boolean moveStatus = myPainter.canMove();
System.out.println("Painter can move forward: " + moveStatus);
Output
Painter can move forward: true
canMove
public boolean canMove(String direction)
Returns true
if there is no barrier one space ahead in the specified direction.
Parameters
Name | Type | Description |
---|---|---|
direction | String | the direction to check |
Examples
canMove("south") Returns false
Painter myPainter = new Painter(2, 3, "east", 0);
boolean moveStatus = myPainter.canMove("south");
System.out.println("Painter can move south: " + moveStatus);
Output
Painter can move south: false
canMove("south") Returns true
Painter myPainter = new Painter(2, 3, "east", 0);
boolean moveStatus = myPainter.canMove("south");
System.out.println("Painter can move south: " + moveStatus);
Output
Painter can move south: true
isOnPaint
public boolean isOnPaint()
Returns true
if there is paint on the space the Painter
object is currently standing on.
Examples
isOnPaint() Returns true
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter.paint("white");
boolean onPaintStatus = myPainter.isOnPaint();
System.out.println("Painter is on paint: " + onPaintStatus);
Output
Painter is on paint: true
isOnPaint() Returns false
Painter myPainter = new Painter(2, 4, "South", 10);
myPainter.paint("white");
myPainter.move();
boolean onPaintStatus = myPainter.isOnPaint();
System.out.println("Painter is on paint: " + onPaintStatus);
Output
Painter is on paint: false
isOnBucket
public boolean isOnBucket()
Returns true
if there is a paint bucket on the space the Painter
object is currently standing on and the paint bucket has paint in it.
Examples
isOnBucket() Returns true
Painter myPainter = new Painter();
myPainter.move();
boolean status = myPainter.isOnBucket();
System.out.println("Painter is on a paint bucket: " + status);
Output
Painter is on a paint bucket: true
isOnBucket() Returns false
Painter myPainter = new Painter();
myPainter.move();
myPainter.move();
boolean status = myPainter.isOnBucket();
System.out.println("Painter is on a paint bucket: " + status);
Output
Painter is on a paint bucket: false
hasPaint
public boolean hasPaint()
Returns true
if the Painter
object has paint in their paint bucket.
Examples
hasPaint() Returns true
Painter myPainter = new Painter(2, 4, "south", 10);
boolean result = myPainter.hasPaint();
System.out.println("Painter has paint: " + result);
Output
Painter has paint: true
hasPaint() Returns false
Painter myPainter = new Painter();
boolean result = myPainter.hasPaint();
System.out.println("Painter has paint: " + result);
Output
Painter has paint: false
isFacingNorth
public boolean isFacingNorth()
Returns true
if the Painter
object is currently facing "North"
.
Examples
isFacingNorth()
returns true
isFacingSouth
public boolean isFacingSouth()
Returns true
if the Painter
object is currently facing "South"
.
Examples
isFacingSouth()
returns true
isFacingEast
public boolean isFacingEast()
Returns true
if the Painter
object is currently facing "East"
.
Examples
isFacingEast()
returns true
isFacingWest
public boolean isFacingWest()
Returns true
if the Painter
object is currently facing "West"
.
Examples
isFacingWest()
returns true
getMyPaint
public int getMyPaint()
Returns the number of units of paint that the Painter
object has in their paint bucket.
Examples
Painter myPainter = new Painter(2, 4, "south", 10);
int paintAmount = myPainter.getMyPaint();
System.out.println("Painter has " + paintAmount + " units of paint.");
Output
Painter has 10 units of paint.
getColor
public String getColor()
Returns the color of the space the Painter
object is currently standing on.
Examples
Painter myPainter = new Painter(2, 4, "south", 10);
myPainter.paint("white");
String currentPaintColor = myPainter.getColor();
System.out.println("Painter is standing on " + currentPaintColor + " paint.");
Output
Painter is standing on white paint.
getX
public int getX()
Returns the x coordinate for the current position of the Painter
object.
Examples
int currentXLocation= myPainter.getX();
System.out.println("Painter is at x location" + currentXLocation);
Output
Painter is at x location 2
getY
public int getY()
Returns the y coordinate for the current position of the Painter
object.
Examples
int currentYLocation= myPainter.getY();
System.out.println("Painter is at y location " + currentYLocation);
Output
Painter is at y location 4
getDirection
public String getDirection()
Returns the direction that the Painter
object is currently facing.
Examples
String currentDirection = myPainter.getDirection();
System.out.println("Painter is facing " + currentDirection);
Output
Painter is facing north
setPaint
public void setPaint(int paint)
Sets the number of units of paint in the Painter
object's paint bucket. If the value passed is a negative number, nothing happens.
Parameters
Name | Type | Description |
---|---|---|
paint | int | the number of units of paint that should be in the |
Examples
Painter myPainter = new Painter(0);
int paintAmount = myPainter.getMyPaint();
System.out.println("Painter has " + paintAmount + " units of paint.");
Output
Painter has 0 units of paint.
myPainter.setPaint(10);
int paintAmount = myPainter.getMyPaint();
System.out.println("Painter has " + paintAmount + " units of paint.");
Output
Painter has 10 units of paint.