penColor
Let the artist in you come out by utilizing the wide range of colors that the turtle can draw with. The color parameter must be a string enclosed in quotes, and can take one of four forms. It can be:
- the name of the color
- the hex value of the color (preceded by a #)
- the rgb value of the color
- the rgba value of the color (last value specifies the alpha channel for transparency)
The default pen color is black and the pen is by default in the down (drawing) position.
Examples
Basic Example
// Sets the color of the line the turtle draws behind it to cyan.
penColor("cyan");
moveForward();
Example: 4 Ways
Demonstrate all 4 ways to specify the color parameter.
// Demonstrate all four ways to specify the color parameter.
// Sets the color using the name of a color in a string.
penColor("chartreuse");
moveForward(50);
turnRight();
// Sets the color using the hex value of a color in a string.
penColor("#7fff00");
moveForward(50);
turnRight();
// Sets the color using the rgb value of a color in a string.
penColor("rgb(127, 255, 0)");
moveForward(50);
turnRight();
// Sets the color using a rgba value of a color in a string.
// The last value is the amount of transparency, a percentage between 0.0 and 1.0
penColor("rgba(127, 255, 0, 0.5)");
moveForward(50);
turnRight();
Example: Four Color Square Spiral
Draw a square spiral using four colors from an array.
// Draw a square spiral using four colors from an array.
var colors = ["red", "magenta", "pink", "purple"];
for (var i = 0; i < 40; i++) {
penColor(colors[i%4]); // Choose a color from the array.
moveForward(100-2*i);
turnRight();
}
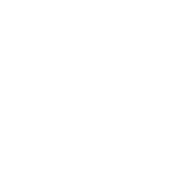
Syntax
penColor(color);
Parameters
Name | Type | Required? | Description |
---|---|---|---|
color | String | The color of the pen used by the turtle for drawing lines and dots. |
Returns
Tips
penUp()
causes no line to be drawn.- Turtle drawing commands are not effected by the
show()
andhide()
commands, which control if the turtle icon is displayed or not. - Recall Unit 1 lessons about hex and rgb color values and see HTML named colors for a complete list of all available colors.
- To randomize color selection, use
penRGB(r,g,b)
which takes numeric parameters instead of a string parameter.
Found a bug in the documentation? Let us know at support@code.org.