turnRight
turnRight() is one of the ways to change the turtle's orientation. When used with moveForward(), you can move, and draw with, the turtle anywhere on the screen.
Examples
Example: Turn Right
// Rotate the turtle right 90 degrees (the default angle) from the current direction.
turnRight();
Example: Step
Draw a step with a right turn and a left turn.
// Draw a step with a right turn and a left turn.
moveForward();
turnRight();
moveForward();
turnLeft();
Example: Letter W
Draw the letter W with right turns only.
// Draw the letter W with right turns only.
turnRight(150);
moveForward();
turnRight(-120);
moveForward();
turnRight(120);
moveForward();
turnRight(-120);
moveForward();
Example: Star
Draw a 25 pointed star by first calculating the exterior angle turn necessary.
var points = 25;
var exteriorAngle = 180.0 - (180.0 / points);
for (var i = 0; i < points; i++) {
moveForward(200);
turnRight(exteriorAngle);
}
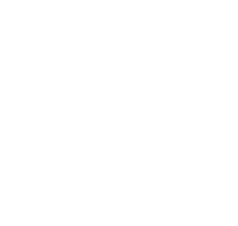
Syntax
turnRight(angle);
Parameters
Name | Type | Required? | Description |
---|---|---|---|
angle | number | The angle to rotate right (90 degrees is default). |
Returns
Tips
- You can specify a negative angle to turn right, which makes the turtle turn left instead.
- There are three ways to rotate the turtle in place
- turnRight(angle) - Rotates the turtle right by the specified angle relative to the current turtle direction. The turtle’s position remains the same.
- turnLeft(angle) - Rotates the turtle left by the specified angle relative to the current turtle direction. The turtle’s position remains the same.
- turnTo(angle) - Rotates the turtle to a specific angle. 0 is up, 90 is right, 180 is down, and 270 is left. The turtle’s position remains the same.
Found a bug in the documentation? Let us know at support@code.org.